Course Overview:
Welcome to the “TypeScript Fundamentals” course, a comprehensive program designed to equip developers, both novices and experienced, with the skills to leverage the power of TypeScript for building robust and scalable web applications. TypeScript, a superset of JavaScript, introduces strong typing and additional features to enhance the development process. This course will guide you through TypeScript’s core concepts and advanced features, empowering you to create maintainable and efficient code.
Course Objectives:
- Introduction to TypeScript:
- Overview of TypeScript and its role in web development
- Setting up a TypeScript development environment
- Understanding TypeScript’s relationship with JavaScript
- TypeScript Basics:
- Variable declarations and data types
- Functions and parameterized types
- Interfaces and type aliases for defining structures
- Advanced Type Features:
- Union and intersection types
- Generics for creating reusable components
- Conditional types for dynamic type expressions
- Working with Classes and Interfaces:
- Creating classes and interfaces in TypeScript
- Implementing inheritance and abstract classes
- Utilizing access modifiers for encapsulation
- Modules and Namespaces:
- Organizing code with modules
- Importing and exporting modules in TypeScript
- Understanding namespaces and their applications
- Advanced TypeScript Configuration:
- tsconfig.json and compiler options
- Strict mode and its benefits
- Using declaration files for external libraries
- Working with Asynchronous Code:
- Promises and async/await in TypeScript
- Handling asynchronous operations effectively
- Error handling and exception patterns
- Building Web Applications with TypeScript:
- Integrating TypeScript with popular front-end frameworks (Angular, React)
- Using TypeScript in Node.js environments
- Debugging and profiling TypeScript applications
Real-world Applications and Case Studies:
- Applying TypeScript concepts in real-world scenarios
- Analyzing successful TypeScript-based applications
- Collaborative coding exercises and hands-on applications
Assessment and Certification:
- Quizzes and coding assignments after each module
- Developing a complete TypeScript project as a final assessment
- Course completion certificate
By the end of this course, you’ll have the skills and confidence to leverage TypeScript for building modern, scalable web applications. Join us on this exciting journey to master the fundamentals of TypeScript and elevate your web development capabilities!
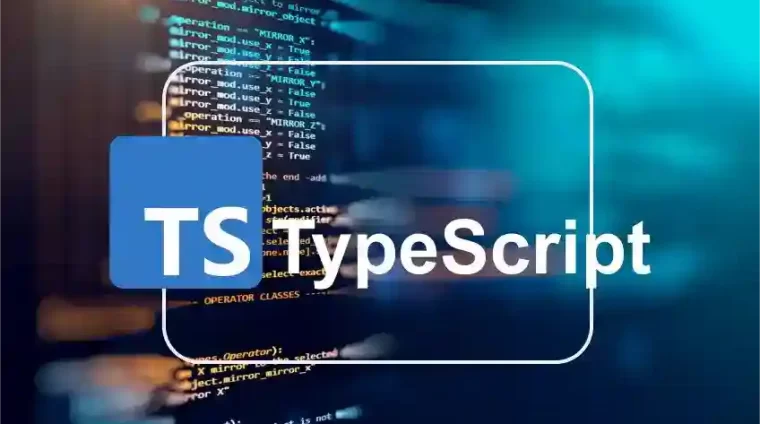
Courses you might be interested in
-
16 Lessons